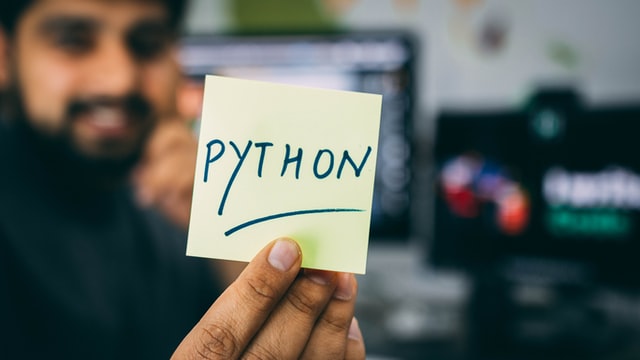
If you have a list of lists, and want to flatten them into one list, there are a few ways to do this. The simplest way is just to iterate through the list and concatenate all the values of each list together.
The flatten list of lists python is a question about how to flatten a list and list of lists in Python.
In Python, lists are one of the most frequently used data types for storing many values in a single variable. A list of Lists is a nested list in which each list element is a list. Converting a two-dimensional list into a single-dimensional list is known as flattening the list of lists.
A list or a list of lists may be flattened in a variety of ways. Let’s look at how to flatten a list or a collection of lists in Python.
Also see: How to make a Python list.
A list of Lists, often known as a 2D list, is a hierarchical list in which each list member is a list. Consider the following scenario:
[[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I list1 = [[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I
In the example above, ‘list1’ is a list, and each element in list1 is a list. As a result, list1 is a collection of lists.
A list has a fixed count, and all of its items are indexed, with zero as the initial index. The index number of each element in a list is used to refer to it.
Lists of lists are divided into two categories: regular and irregular. Each element of a Regular list of lists is a sublist, while each element of an Irregular list of lists may be a sublist or a single element. Consider the following scenario:
[[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I Regular list of lists list1 = [[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I [[‘a’, ‘b’], ‘c’, [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I ‘j’, ‘k’], ‘l’] Irregular list of lists list2 = [[‘a’, ‘b’], ‘c’, [‘d’, ‘e’, ‘f’], [‘g’, ‘h’,
To flatten the list of lists, you may use loops.
Flattening Lists on a regular basis
See the sample code below for an example of how to flatten a normal list of lists using loops.
[[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I list1= [[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I [] final list to be printed (“The original list is: “,list) # use a for loop to go through the data for element in list1: print(“The flatten list is: “, final list) print(“The flatten list is: “, final list) print(“The flatten list is: “, final list) print(“The flatten list is: “, final list) print(“The flatten list
The following is the result of the aforementioned code:
[[‘a’, ‘b’, ‘c’], [‘d’, ‘e’, ‘f’], [‘g’, ‘h’, I is the original list. [‘a’, ‘b’, ‘c’, ‘d’, ‘e’, ‘f’, ‘g’, ‘h’, I is the flatten list.
Creating a flattened irregular list of lists
To learn how to utilize loops to flatten an irregular list of lists, look at the sample code below.
[1, 2, 3, [4, 5], 6, [[7], 8]] list1 [] final list to be printed (“original list is: “, list1) flat list(list1): def flat list(list1): def flat list(list1): # iterate through the list for element in list1 using for loop: if type(element) is equal to list: If the element is a list, flat list(element) is called recursively with the current element as the new argument; otherwise, final list is called. append(element) # using the flat list function to flatten the list (list1) print(“flattened list is: “,final list) # outputting the final list
The following is the result of the aforementioned code:
[1, 2, 3, [4, 5], 6, [[7]. 8]] is the original list. [1, 2, 3, 4, 5, 6, 7, 8] is the flattened list.
NumPy is a Python module that includes a number of methods and functions for working with arrays. You may flatten your list of lists into a list by using the NumPy package’s concatenate() and flat() methods. See the following code for an example:
[[1, 2], [3, 4], [5, 6, 7]] import numpy list1= list(numpy.concatenate); final list = list(numpy.concatenate); (list1). flat) list1) print(‘Original list is:’, print(‘Original list is:’, print(‘Original list is:’, final list = print(‘Flattened list is:’)
The following is the result of the aforementioned code:
[[1, 2], [3, 4], [5, 6. 7]] is the original list. [1, 2, 3, 4, 5, 6, 7] is the flattened list.
The chain function in Python’s Itertools module iterates over the list and returns the items as an iterable, which you must subsequently convert into a list. Take a look at the following code as an example:
to be printed (“Original list is: “,list1) # Using the chain() technique to flatten the list list(itertools.chain(*list1)) = final list “Flattened list is: “,final list) print(“Flattened list is: “,final list) print(“Flattened list
The following is the result of the aforementioned code:
[[1, 2, 3, 4], [5, 6], [7, 8, 9]] is the original list. [1, 2, 3, 4, 5, 6, 7, 8, 9] is the flattened list.
You may flatten your list of lists into a single list using any of the methods listed above.
Also see: Python: How to Find the Length of a List
An enthusiastic reader and a student of engineering. I like coding and reading. I’m always eager to learn new things.
The flatten nested list python is a question that has been asked before. There are two ways to flatten lists in Python, either by using the built-in function list.flatten() or using the built-in function itertools.chain().
Frequently Asked Questions
How do you flatten a list in Python?
How do you flatten a nested list in Python?
How do you recursively flatten a list in Python?
This is not possible in Python.
Related Tags
- list of list to list python
- python flatten list of lists and ints
- flatten a list python
- python flatten list recursive
- flatten list of tuples python